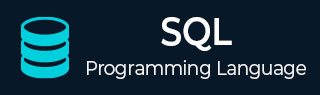
- SQL Tutorial
- SQL - Home
- SQL - Overview
- SQL - RDBMS Concepts
- SQL - Databases
- SQL - Syntax
- SQL - Data Types
- SQL - Operators
- SQL - Expressions
- SQL Database
- SQL - Create Database
- SQL - Drop Database
- SQL - Select Database
- SQL - Rename Database
- SQL - Show Database
- SQL - Backup Database
- SQL Table
- SQL - Create Table
- SQL - Show Tables
- SQL - Rename Table
- SQL - Truncate Table
- SQL - Clone Tables
- SQL - Temporary Tables
- SQL - Alter Tables
- SQL - Drop Table
- SQL - Delete Table
- SQL - Constraints
- SQL Queries
- SQL - Insert Query
- SQL - Select Query
- SQL - Select Into
- SQL - Insert Into Select
- SQL - Update Query
- SQL - Delete Query
- SQL - Sorting Results
- SQL Views
- SQL - Create Views
- SQL - Update Views
- SQL - Drop Views
- SQL - Rename Views
- SQL Operators and Clauses
- SQL - Where Clause
- SQL - Top Clause
- SQL - Distinct Clause
- SQL - Order By Clause
- SQL - Group By Clause
- SQL - Having Clause
- SQL - AND & OR
- SQL - BOOLEAN (BIT) Operator
- SQL - LIKE Operator
- SQL - IN Operator
- SQL - ANY, ALL Operators
- SQL - EXISTS Operator
- SQL - CASE
- SQL - NOT Operator
- SQL - NOT EQUAL
- SQL - IS NULL
- SQL - IS NOT NULL
- SQL - NOT NULL
- SQL - BETWEEN Operator
- SQL - UNION Operator
- SQL - UNION vs UNION ALL
- SQL - INTERSECT Operator
- SQL - EXCEPT Operator
- SQL - Aliases
- SQL Joins
- SQL - Using Joins
- SQL - Inner Join
- SQL - Left Join
- SQL - Right Join
- SQL - Cross Join
- SQL - Full Join
- SQL - Self Join
- SQL - Delete Join
- SQL - Update Join
- SQL - Left Join vs Right Join
- SQL - Union vs Join
- SQL Keys
- SQL - Unique Key
- SQL - Primary Key
- SQL - Foreign Key
- SQL - Composite Key
- SQL - Alternate Key
- SQL Indexes
- SQL - Indexes
- SQL - Create Index
- SQL - Drop Index
- SQL - Show Indexes
- SQL - Unique Index
- SQL - Clustered Index
- SQL - Non-Clustered Index
- Advanced SQL
- SQL - Wildcards
- SQL - Comments
- SQL - Injection
- SQL - Hosting
- SQL - Min & Max
- SQL - Null Functions
- SQL - Check Constraint
- SQL - Default Constraint
- SQL - Stored Procedures
- SQL - NULL Values
- SQL - Transactions
- SQL - Sub Queries
- SQL - Handling Duplicates
- SQL - Using Sequences
- SQL - Auto Increment
- SQL - Date & Time
- SQL - Cursors
- SQL - Common Table Expression
- SQL - Group By vs Order By
- SQL - IN vs EXISTS
- SQL - Database Tuning
- SQL Function Reference
- SQL - Date Functions
- SQL - String Functions
- SQL - Aggregate Functions
- SQL - Numeric Functions
- SQL - Text & Image Functions
- SQL - Statistical Functions
- SQL - Logical Functions
- SQL - Cursor Functions
- SQL - JSON Functions
- SQL - Conversion Functions
- SQL - Datatype Functions
- SQL Useful Resources
- SQL - Questions and Answers
- SQL - Quick Guide
- SQL - Useful Functions
- SQL - Useful Resources
- SQL - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
SQL - Operators
What is an Operator in SQL?
An SQL operator is a reserved word or a character used primarily in an SQL statement's WHERE clause to perform operation(s), such as comparisons and arithmetic operations. These Operators are used to specify conditions in an SQL statement and to serve as conjunctions for multiple conditions in a statement.
An SQL operator can be either a unary or binary operator. A unary operator (example unary + or unary - ) uses only one operand to perform the unary operation, whereas the binary operator (example + or - etc) uses two operands to perform the binary operation.
SQL supports following types of operators:
- Arithmetic operators
- Comparison operators
- Logical operators
- Operators used to negate conditions
SQL Arithmetic Operators
SQL Arithmetic Operators are used to perform mathematical operations on the numerical values. SQL provides following operators to perform mathematical operations.
Here is a list of all the arithmetic operators available in SQL.
Operator | Description | Example |
---|---|---|
+ | Adds the values both sides of the operator. | 10 + 20 gives 30 |
- | Subtracts right hand operand from left hand operand. | 20 - 30 gives -10 |
* | Multiplies the values from both sides of the operator. | 10 * 20 gives 200 |
/ | Divides left hand operand by right hand operand. | 20 / 10 gives 2 |
% | Divides left hand operand by right hand operand and returns the reminder remainder. | 5 % 2 gives 1 |
SQL Comparison Operators
SQL Comparison Operators test whether two given expressions are the same or not. These operators are used in SQL conditional statements while comparing one expression with another and they return a Boolean value which can be either TRUE or FALSE. The result of an SQL comparison operatoration can be UNKNOWN when one or another operand has it’s value as NULL.
Here is a list of all the comparison operators available in SQL.
Operator | Description | Example |
---|---|---|
= | Checks if the values of two operands are equal or not, if yes then condition becomes true. | 5 = 5 returns TRUE |
!= | Checks if the values of two operands are equal or not, if values are not equal then condition becomes true. | 5 != 6 returns TRUE |
<> | Checks if the values of two operands are equal or not, if values are not equal then condition becomes true. | 5 <> 4 returns TRUE |
> | Checks if the value of left operand is greater than the value of right operand, if yes then condition becomes true. | 4 > 5 returns FALSE |
< | Checks if the value of left operand is less than the value of right operand, if yes then condition becomes true. | 4 < 5 returns TRUE |
>= | Checks if the value of left operand is greater than or equal to the value of right operand, if yes then condition becomes true. | 4 >= 5 returns FALSE |
<= | Checks if the value of left operand is less than or equal to the value of right operand, if yes then condition becomes true. | 4 <= 5 returns TRUE |
!< | Checks if the value of left operand is not less than the value of right operand, if yes then condition becomes true. | 4 !< 5 returns FALSE |
!> | Checks if the value of left operand is not greater than the value of right operand, if yes then condition becomes true. | 4 !> 5 returns TRUE |
SQL Logical Operators
SQL Logical Operators are very similar to comparison operators and they test for the truth of some given condition. These operators return a Boolean value which can be either a TRUE or FALSE. The result of an SQL logical operatoration can be UNKNOWN when one or another operand has it’s value as NULL.
Here is a list of all the logical operators available in SQL.
Operator | Description | Example |
---|---|---|
ALL | TRUE if all of a set of comparisons are TRUE. | |
AND | TRUE if all the conditions separated by AND are TRUE. | |
ANY | TRUE if any one of a set of comparisons are TRUE. | |
BETWEEN | TRUE if the operand lies within the range of comparisons. | |
EXISTS | TRUE if the subquery returns one or more records | |
IN | TRUE if the operand is equal to one of a list of expressions. | |
LIKE | TRUE if the operand matches a pattern specially with wildcard. | |
NOT | Reverses the value of any other Boolean operator. | |
OR | TRUE if any of the conditions separated by OR is TRUE | |
IS NULL | TRUE if the expression value is NULL. | |
SOME | TRUE if some of a set of comparisons are TRUE. | |
UNIQUE | The UNIQUE operator searches every row of a specified table for uniqueness (no duplicates). |
SQL Operators Precedence
The operators precedence in SQL is the sequence in which the SQL evaluates the different operators in a given expression. The operators with higher precedence get evaluated first.
Following table lists all SQL operators as per their precedence. The operators with the highest precedence are at the top and the operators with the lowest precedence are at the bottom.
Operator | Operation |
---|---|
+, - | identity, negation |
*, / | multiplication, division |
+, - | addition, subtraction |
=, !=, <, >, <=, >=, IS NULL, LIKE, BETWEEN, IN | Comparison |
NOT | logical negation |
AND | conjunction |
OR | inclusion |
Example
Consider the following SQL statement:
SELECT 20 - 3 * 5
This will result in the following. Here multiplication operator gets evaluated first and then subtraction happens.
5