
- Python Basic Tutorial
- Python - Home
- Python - Overview
- Python - Environment Setup
- Python - Basic Syntax
- Python - Comments
- Python - Variables
- Python - Data Types
- Python - Operators
- Python - Decision Making
- Python - Loops
- Python - Numbers
- Python - Strings
- Python - Lists
- Python - Tuples
- Python - Dictionary
- Python - Date & Time
- Python - Functions
- Python - Modules
- Python - Files I/O
- Python - Exceptions
- Python Advanced Tutorial
- Python - Classes/Objects
- Python - Reg Expressions
- Python - CGI Programming
- Python - Database Access
- Python - Networking
- Python - Sending Email
- Python - Multithreading
- Python - XML Processing
- Python - GUI Programming
- Python - Further Extensions
- Python Useful Resources
- Python - Questions and Answers
- Python - Quick Guide
- Python - Tools/Utilities
- Python - Useful Resources
- Python - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to Python. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
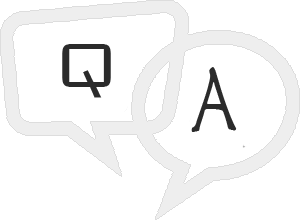
Q 1 - What is the output for −
'Tutorials Point' [100:200]?
Answer : B
Explanation
Slicing will never give us an error even if we give it too large of an index for the string. It will just return the widest matching slice it can.
Answer : A
Explanation
z = z++ is not valid in python, it is not a legal expression. It results in syntax error.
Q 3 - Pylab is a package that combine _______,________&______ into a single namespace.
B - Numpy, matplotlib & pandas
Answer : A
Explanation
pylab package in python combines numpy, scipy & matplotlib into a single namespace.
Q 4 - Suppose we have two sets A & B, then A<B is:
A - True if len(A) is less than len(B).
B - True if A is a proper subset of B.
C - True if the elements in A when compared are less than the elements in B.
Answer : B
Explanation
If A is proper subset of B then hen all elements of A are in B but B contains at least one element that is not in B.
Q 5 - Find the output of the code?
def f(a, b = 1, c = 2): print('a is: ',a, 'b is: ', b, 'c is: ', c) f(2, c = 2) f(c = 100, a = 110)
a is: 110 b is: 1 c is: 100
a is: 110 b is: 2 c is: 100
a is: 110 b is: 0 c is: 100
D - a is: 110 b is: 0 c is: 100
a is: 110 b is: 0 c is: 100
Answer : A
Explanation
Value of Arguments is passed to the parameters in order of their sequence.
Q 6 - Which of the following is more accurate for the following declaration?
x = Circle()
A - Now you can assign int value to x.
B - x contains a reference to a Circle object.
Answer : B
Explanation
x is the object created by the constructor of the class Circle().
Q 7 - What is the out of the code?
def rev_func(x,length): print(x[length-1],end='' '') rev_func(x,length-1) x=[11, 12, 13, 14, 15] rev_func(x,5)
A - The program runs fine without error.
B - Program displays 15 14 13 12 11.
C - Program displays 11 12 13 14 15.
D - Program displays 15 14 13 12 11 and then raises an index out of range exception.
Answer : D
Explanation
In the print statement of rev_func we use the index value of list x. We decrement the value of length of function because it becomes the index of x in the print statement.
Q 8 - Which function can be used on the file to display a dialog for saving a file?
B - Filename = asksavefilename()
Answer : C
Explanation
This is the default method to display a dialog for saving a file in Tkinter module of Python.
Q 9 - Suppose you are using a grid manager then which option is best suitable to place a component in multiple rows and columns?
Answer : A
Q 10 - How you can lift the pen of in turtle?