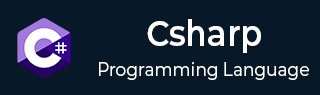
- C# Basic Tutorial
- C# - Home
- C# - Overview
- C# - Environment
- C# - Program Structure
- C# - Basic Syntax
- C# - Data Types
- C# - Type Conversion
- C# - Variables
- C# - Constants
- C# - Operators
- C# - Decision Making
- C# - Loops
- C# - Encapsulation
- C# - Methods
- C# - Nullables
- C# - Arrays
- C# - Strings
- C# - Structure
- C# - Enums
- C# - Classes
- C# - Inheritance
- C# - Polymorphism
- C# - Operator Overloading
- C# - Interfaces
- C# - Namespaces
- C# - Preprocessor Directives
- C# - Regular Expressions
- C# - Exception Handling
- C# - File I/O
- C# Advanced Tutorial
- C# - Attributes
- C# - Reflection
- C# - Properties
- C# - Indexers
- C# - Delegates
- C# - Events
- C# - Collections
- C# - Generics
- C# - Anonymous Methods
- C# - Unsafe Codes
- C# - Multithreading
- C# Useful Resources
- C# - Questions and Answers
- C# - Quick Guide
- C# - Useful Resources
- C# - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C# Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C#. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
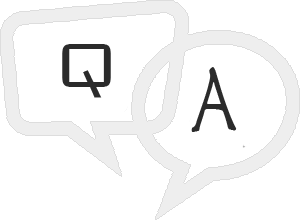
Answer : B
Explanation
Keywords are reserved words predefined to the C# compiler. These keywords cannot be used as identifiers.
Q 2 - Which of the following is correct about reference type variables in C#?
A - The reference types do not contain the actual data stored in a variable.
B - They contain a reference to the variables.
C - Example of built-in reference types are: object, dynamic, and string.
Answer : D
Explanation
All of the above options are correct.
Q 3 - Which of the following converts a type to a 16-bit integer in C#?
Answer : C
Explanation
ToInt16() method converts a type to a 16-bit integer.
Q 4 - Which of the following operator represents a conditional operation in C#?
Answer : A
Explanation
?: operator represents a conditional operation.
Q 5 - Which of the following access specifier in C# allows a child class to access the member variables and member functions of its base class?
Answer : C
Explanation
Protected access specifier allows a child class to access the member variables and member functions of its base class.
Q 6 - Which of the following is correct about params in C#?
B - Additional parameters are not permitted after the params keyword in a method declaration.
C - Only one params keyword is allowed in a method declaration.
Answer : D
Explanation
All of the above statements are correct.
Q 7 - Which of the following is the correct about class member functions?
Answer : C
Explanation
Both of the above options are correct.
Q 8 - Which of the following is the correct about static member functions of a class?
A - You can also declare a member function as static.
B - Such functions can access only static variables.
C - The static functions exist even before the object is created.
Answer : D
Explanation
All of the above options are correct.
Q 9 - Which of the following preprocessor directive defines a sequence of characters as symbol in C#?
Answer : A
Explanation
#define: It defines a sequence of characters, called symbol.
Q 10 - Which of the following is true about catch block in C#?
B - The catch keyword indicates the catching of an exception.
Answer : C
Explanation
Both of the above options are correct.