
- C Programming Tutorial
- C - Home
- C - Overview
- C - Environment Setup
- C - Program Structure
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Constants
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - Loops
- C - Functions
- C - Scope Rules
- C - Arrays
- C - Pointers
- C - Strings
- C - Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Recursion
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming useful Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
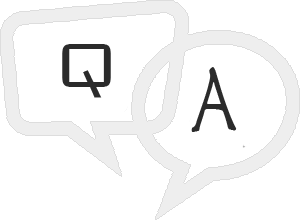
Q 1 - What is the outpout of the following program?
#include<stdio.h> main() { enum { india, is=7, GREAT }; printf("%d %d", india, GREAT); }
Answer : C
Explanation
0 8, enums gives the sequence starting with 0. If assigned with a value the sequence continues from the assigned value.
Q 2 - What is the value of ‘y’ for the following code snippet?
#include<stdio.h> main() { int x = 1; float y = x>>2; printf( "%f", y ); }
Answer : C
Explanation
0, data bits are lost for the above shift operation hence the value is 0.
Answer : B
Explanation
volatile is the reserved keyword and cannot be used an identifier name.
Q 4 - Choose the invalid predefined macro as per ANSI C.
Answer : D
Explanation
There is no macro define with the name __C++__, but __cplusplus is defined by ANSI)
Q 5 - What is the output of the following program?
#include<stdio.h> main() { char s[20] = "Hello\0Hi"; printf("%d %d", strlen(s), sizeof(s)); }
Answer : C
Explanation
Length of the string is count of character upto ‘\0’. sizeof – reports the size of the array.
Answer : A
Explanation
#include <stdio.h> int main() { long int decimalNumber,remainder,quotient; int binaryNumber[100], i = 1, j; printf("Enter any decimal number: "); scanf("%ld",&decimalNumber); quotient = decimalNumber; while(quotient!=0){ binaryNumber[i++]= quotient % 2; quotient = quotient / 2; } printf("Equivalent binary value of decimal number %d: ",decimalNumber); for(j = i -1 ;j> 0;j--) printf("%d",binaryNumber[j]); return 0; }
Q 7 - Which header file supports the functions - malloc() and calloc()?
Answer : A
Explanation
void *malloc(size_t size) : Allocates the requested memory and returns a pointer to it.
void *calloc(size_t nitems, size_t size): Allocates the requested memory and returns a pointer to it.
Q 8 - How many times the given below program will print "IndiaPIN"?
#include<stdio.h> int main () { printf("IndiaPIN"); main(); return 0; }
Answer : D
Explanation
A stack over flow comes when over loaded memory is used by the call stack. Here, main()function is called repeatedly and its return address stores in the stack. When stack memory get filled, it displays the error “stack overflow”.
#include<stdio.h> int main () { printf("IndiaPIN"); main(); return 0; }
Q 9 - What will be the output of the following program?
#include<stdio.h> int main() { const int x = 5; const int *ptrx; ptrx = &x; *ptrx = 10; printf("%d\n", x); return 0; }
Answer : D
Explanation
The above program will return error
#include<stdio.h> int main() { const int x = 5; const int *ptrx; ptrx = &x; *ptrx = 10; printf("%d\n", x); return 0; }
Q 10 - The library function strrchr() finds the first occurrence of a substring in another string.
Answer : B
Explanation
Strstr() finds the first occurrence of a substring in another string.